What is Docker?
Docker has gained its popularity for multiple reasons, one of them is creating portable containers which are fast & easy to deploy. As mentioned on their website a container packages your code along with any other dependencies so that it can be deployed across multiple platforms reliably. These containers can be run locally on your Windows, Mac & Linux. Secondly major cloud systems like AWS or Azure do support them out of the box. Lastly, it can be used on any hosting space where it can be installed and run.
Containers are the building blocks of docker. In this blog we will go through various commands that will help us manipulate and create containers. As we go through each command you will have a better understanding of how docker works. So let’s stick through till the end. 🙂
Prerequisites
Getting Started
Execute below command to verify docker installation:
docker --version
It will print the version installed. Now, we will create a very basic nodejs app to containerize.
Create a folder named yay-docker. Secondly, create two files named server.js and package.json as below:
{
"name": "yay-docker",
"dependencies": {
"express": "^4.17.1"
}
}
const server = require("express")();
server.listen(8080, async () => { });
server.get("/yay-docker", async (_, response) => {
console.log('Request Received for yay-docker');
response.json({ "yay": "docker" });
});
In order create a container we first need to create a “Dockerfile” as below:
FROM node:17-slim
WORKDIR /app
COPY . .
RUN npm install
CMD ["node", "server.js"]
EXPOSE 3000
That is our basic setup from which we will create an image and run it as a container.
Essential Docker Commands
We will be looking at the below functionalities of docker. For that, navigate to your project directory from your terminal or command prompt.
Create an image
docker build -t yay-docker .
Run the above command to start creating an image of your project. Here, yay-docker is the name of image that you want to create. It will start multiple layers for downloading the node:17-slim image. Once done it will run npm install to install dependencies like express from your package.json file. Below is a screen shot after running the command successfully.
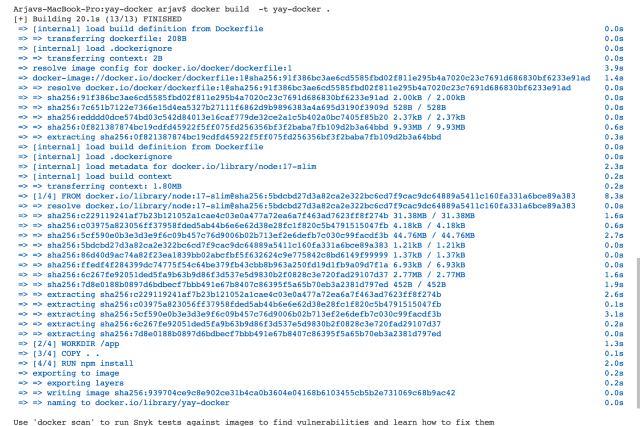
Docker Build
The image is also visible in Docker Desktop software as you can see in the image below.

Images in Docker Desktop
Run the image as container
docker run -p 3000:8080 -d yay-docker
The above command will simply run the image named yay-docker. 3000 is the container’s port number. While 8080 is the port number of the node app running in our container. You can view the list of containers in the docker desktop app as seen in the screenshot below.
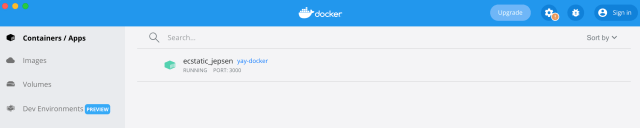
List of Containers
To test if your container is running successfully open http://localhost:3000/yay-docker on your browser. A nice json text {“yay”:”docker”} will be displayed. Congratulations! you have successfully run your first container.
List Containers
docker ps -a
Docker Desktop displays the list of containers if running locally. But incase if there is a need to see via command line, you can fire the above command. It will list down all containers along with the container id, it’s up time and the status. The container id is used to manipulate the container as we will see below
Container Logs
docker logs <container-id>
What if you want to see what your application is printing in the console. The above command does exactly that. It prints the logs of your container.
In the above command replace the <container-id> with the id from the list of containers. You can see a line/s saying “Request Received for yay-docker” in the logs. This is usually helpful when debugging on the cloud for any errors or checking the console logs.
Entering the Container
docker exec -it <container-id> /bin/sh
At times you would like to enter into the container to collect information. e.g. viewing logs files. It can be easily done with the above command. You can type ls command to view the list files in the container’s /app directory and manipulate the container as you want.
Enter exit command to exit from the container.
Bonus Commands
Start Container
docker start <container-id>
Stop Container
docker stop <container-id>
Remove Container
docker rm <container-id>
Where to go from here
Hope you have gained a good insight on what Docker is and how to containerise your application for better stability. While a Part 2 is in the works you can visit the official documentation for understanding more advanced feature set.
You can file the whole project on my Github account: https://github.com/shenanigan/yay-docker
In the next part we will under stand what docker compose is along with some exciting new things we can do with it.
I am sure you will love my other articles:
Comments